How to Draw the Australian Flag
12 Nov
This is one a series of posts on how to draw world flags. Finding the specifications for these flags, and attempting to reproduce the flag perfectly, according to the specifications, is a great way to teach yourself basic graphics programming.
Previously: Union Jack
Okay, in previously tutorials I have covered all the ingredients that go into the Australian flag, specifically:
Here’s my sketch of flag of Australia, the specification of which you’ll find in Wikipedia.
So, first, let’s talk about colors. Although the Union Jack of the United Kingdom looks very similar, color-wise, the Australian government has specified different colors for this flag. They recommend both Pantone colors, for physical flags, and RGB colors for flags on websites. Here you can see what both look like:
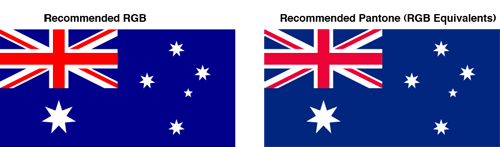
I don’t particularly like the government recommended RGB colors, which I find to be over-saturated. So I decided to go-against the Australian government and use RGB equivalents of their recommended Pantone colors. I think they look nicer. You can use whatever colors you want – I provided both sets in the sketch. Just for reference, here’s what the Union Jack recommended colors look like:
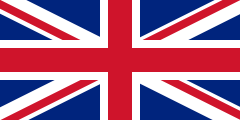
Now onto the Union Jack. My first thought was to take my existing Union Jack code, and drop it into a function, which takes w and h (width and height) as arguments, so I can draw a Union Jack any size. Then I would pass in width/2 and height/2 to get the correctly sized Union Jack. This would have worked except for one problem, as you can see in the following buggy flag:
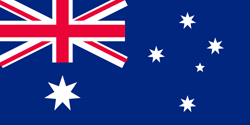
If you look closely, you’ll see the white and red diagonal stripes extend too far out, and aren’t cropped to the square. This is due to the lazy way I originally implemented the Union Jack – I drew those stripes as thick lines and allowed the frame of the sketch to crop them out.
I could have fixed this by changing the way I drew the lines, drawing them as quadrilateral outlines, rather than thick lines. This would have worked, but I decided to go another route, so I could demonstrate how to draw into an offscreen bitmap.
What I did was to create an offscreen PGraphics object which is the size I want, and then I draw the Union Jack into that. This causes the diagonal stripes to be cropped to the size of the PGraphics object, which is one quarter the area of my main window. Then, I copy that PGraphics image into my larger drawing window.
Here’s the code to set it up.
unionJack = createGraphics(width/2, height/2, P2D); unionJack.beginDraw(); drawUnionJack(unionJack); unionJack.endDraw();
The drawUnionJack() function that I created is essentially the same code from my Union Jack tutorial, but modified to draw into the offscreen buffer. Take a look at the complete source code, to view it.
To draw the Australian flag, I first fill the background with my blue color, then I copy my previously prepared Union Jack using the image() function to draw it at 0,0:
background(blueColor); image(unionJack, 0, 0);
Then I draw my stars, using the drawAster() function I covered in the previous tutorial on drawing stars. The Australian Flag specification on Wikipedia specifies precisely how big these stars are (both inner and outer radii) and where they are placed. I’ve duplicated those measurements here.
// Commonwealth star drawAster( 7, width/4.0, height*3/4.0, width*3/20.0, 4/9.0); // Alpha Crucis drawAster( 7, width*3/4.0, height*5/6.0, width*1/14.0, 4/9.0); // Beta Crucis drawAster( 7, width*5/8.0, height*7/16.0, width*1/14.0, 4/9.0); // Gamma Crucis drawAster( 7, width*3/4.0, height*1/6.0, width*1/14.0, 4/9.0); // Delta Crucis drawAster( 7, width*31/36.0, height*89/240.0, width*1/14.0, 4/9.0); // Epsilon Crucis drawAster( 5, width*4/5.0, height*13/24.0, width*1/24.0, 4/9.0);
And voila, we have an Australian flag! Insert marsupial jokes here. At this point, I would suggest trying to draw the American Flag, using the techniques I’ve covered so far (the only tricky part is tiling the stars right). I’ll show you my method in the next flag tutorial.
Tags: intermediate, stars
No comments yet